Simplest JS promise tutorial using await / async
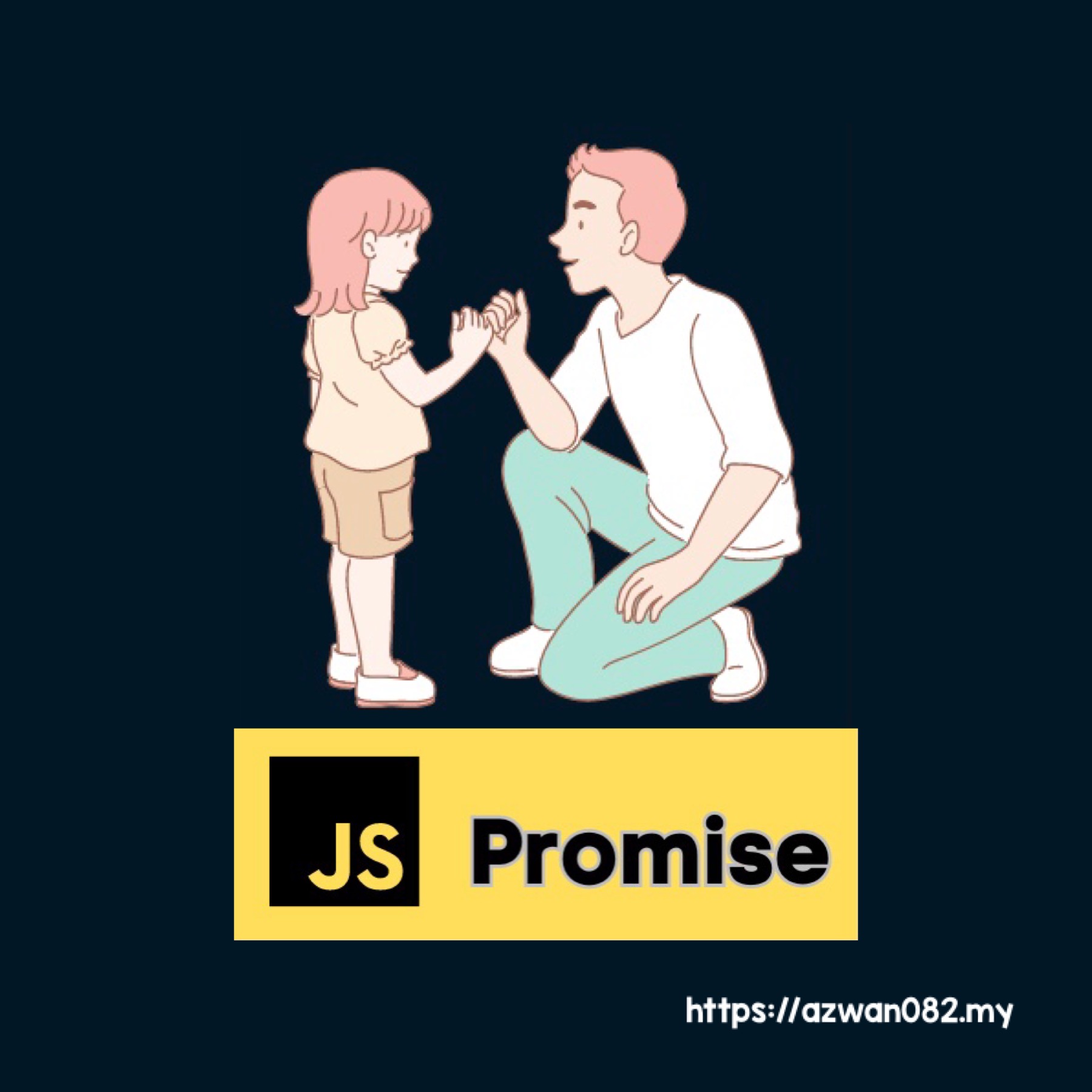
Rabu, 24 April 2024, 4:30 pm
I often forgot how to use output of a function that uses Promise. Which callback to use? .then()
/ .catch()
/ .finally()
/ .all()
?
So here’s the easiest way to use it & remember.
Photos from:
- https://www.freecodecamp.org/news/javascript-promise-tutorial-how-to-resolve-or-reject-promises-in-js/
- https://medium.com/from-code-to-beyond/stop-using-promise-all-in-javascript-a8157bc692bd
26 April 2024
Connection timed out when running commands in macOS
23 April 2024